React – Pass Data From Child to Parent and Parent to Child Component
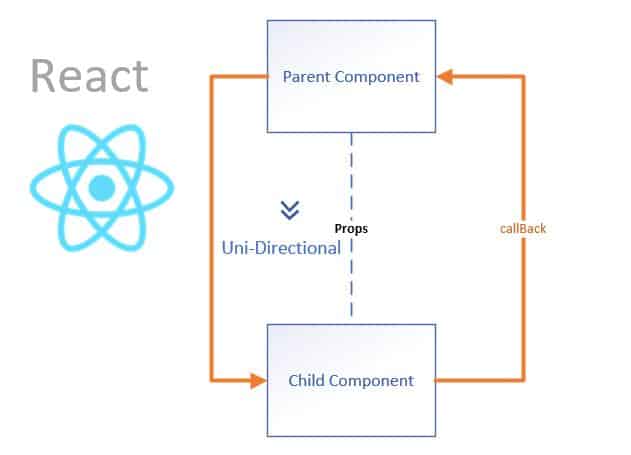
Today in this article we shall learn how to perform component interactions in React UI framework application. You may find a need to Pass Data From One Component to another Component including React – Pass Data From One Component to Other Component with Child to Parent Component from parent to child communication
We shall cover below basic aspects in today’s article,
Before we get started I am assuming you already have a basic understanding of React applications.
If not, kindly go through a series of articles on React,
Getting Started
Let’s get started step by step from basics to advanced topics.
What is Props System
Props are actually a short form of Properties. It’s a mechanism of passing the data from Parent components to child components. Using Props data must flow uni-directionally.
Using Props data must flow uni-directionally i.e Parent to Child only
This data transfer happens in two stages. In the first stage, we provide information from the parent to the child components.

So let’s first focus on how we provide information from the parent to the child and then in the second stage we will see how the Child Component consumes or makes use of that information.
I shall be using very basic two components defined as below,
- Parent Component – UserComponent
- Child Component – MovieComponent
Create Parent Component – UserComponent
Let’s create a simple Parent Component – UserComponent as below,
class UserComponent extends React.Component {
render() {
return (
<div className="content">
Hi There, UserComponent
</div>
);
}
}
export default UserComponent;
Create Child Component – MovieComponent
Similarly, Let’s create a simple Child Component – MovieComponent as below,
class MovieComponent extends React.Component {
render() {
return (
<div className="content">
Hi There, MovieComponent
</div>
);
}
}
export default MovieComponent;
Now, these components are currently independent and there is no parent-child relationship so far,
Below is index.json referencing them individually,
ReactDOM.render(
<React.StrictMode>
<UserComponent/>
<MovieComponent/>
</React.StrictMode>,
document.getElementById('root')
);
Once you launch the app, you shall see both components execute and produce the below result,
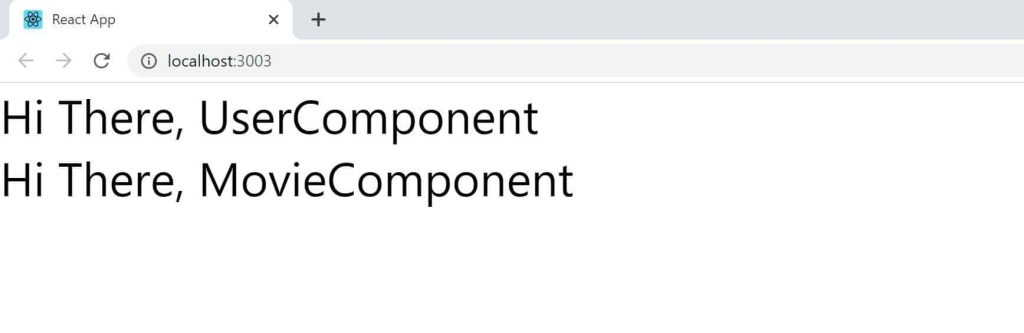
So far so good !!
Let’s now define the relationship which Parent to Child relationship!
Naming Pattern for Props
Before doing that let’s understand the naming convention and patterns that needs to be used while using props in the component.

Lets’ see a few examples,
Example,
<ChildComponent userName ="TheCodeBuzz"/>
<MovieComponent movieName ="Animators"/>
React Parent to Child Component using Props
Let’s update the above-discussed component for Parent to Child communication. Our goal here would be to transfer data from Parent Component UserComponent to the Child component i.e MovieComponent
Update code on Parent as below,
class UserComponent extends React.Component {
render() {
return (
<div className="content">
<div> Hi There, UserComponent</div>
<MovieComponent movie="Animators" />
</div>
);
}
}
export default UserComponent;
So what we did above as below,
- Above we have defined our Props name as movie to pass the data to the child component.
- UserComponent should be able to pass the movie object which has a value of “Animators” defined using the expression MovieComponent movie=”Animators”
Similarly, we shall be retrieving the same Props i.e movie in the Child component as below,
class MovieComponent extends React.Component {
render() {
return (
<div className="content">
<div>Hi There, MovieComponent</div>
Movie selected by User: {this.props.movie}
</div>
);
}
}
export default MovieComponent;
So what we did above as below,
- Child consumes or makes use of Parent information using Props
- Retrieves the same Props i.e movie in the Child component
Once executed, the app shall below result on the UI,

That’s All!
I have discussed Child to Parent communication in the below article,
References
I shall be covering Redux Architecture and its usage in my next article! Until then stay tuned!
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
Today in this article we understood how Component interacts in React programming. We understood the prop system and understood the naming pattern and used it for passing data from Parent to Child.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.